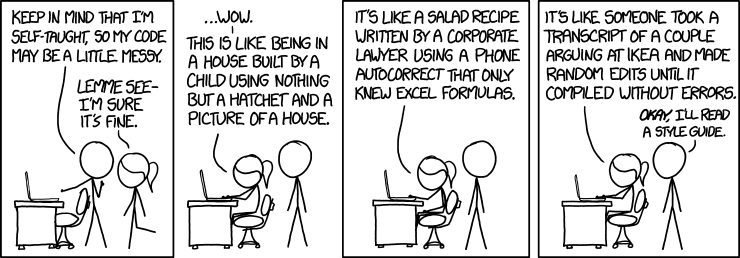
This is our playbook, there are many like it, but this one is ours.
Wanna work with us? We have a fun assigment that you might be interested in.
Email us your GitHub repo!
If your guideline applies to both Swift and Objective-C, then it's probably a best practice.