This repository presents updated and modernized solutions to programming challenges found in Harry He's "Coding Interviews" book, which is a well-regarded resource for candidates preparing for coding interviews. The book can be purchased on Amazon here.
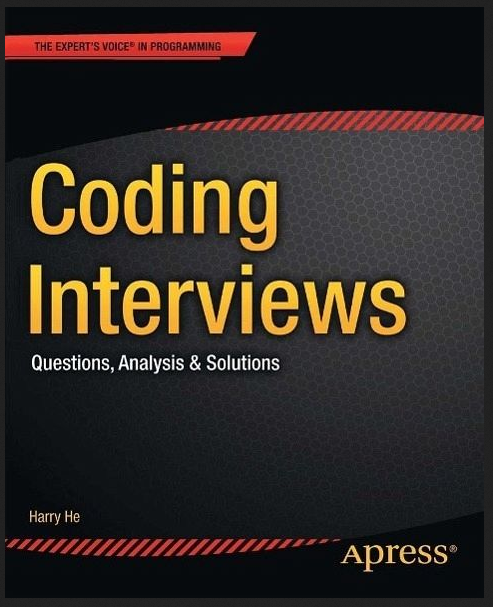
Here are some specific aspects I focused on while modernizing the solutions:
- Error Handling: Replaced error codes with exceptions to handle errors more gracefully.
- Input/Output: Used
iostream
instead of stdlib.h
for standard input and output operations.
- Testing: Swapped console tests with assertions to validate the solutions.
- Data Structures: Preferred using vectors over bare arrays for flexibility.
- Looping: Used iterators instead of integer counters for traversing collections.
- Class Implementation: Implemented collections using classes to encapsulate data.
- References: Used references instead of pointers where possible for safety and readability.
- Functional Programming: Employed lambdas for implementing private functions.
- Simplifying Data: Used STL pairs instead of creating custom structs.
- Type Deduction: Utilized the
auto
keyword for cleaner code and type deduction.
While I've endeavored to improve the solutions, it is important to note that:
- This repository does not provide solutions to every problem mentioned in the book.
- Some solutions in this repository may not correspond to problems directly from the book but are inspired by similar challenges.
# |
Title |
Solution |
1 |
Array rotation |
C++ |
2 |
Duplicates |
C++ |
3 |
Majority element |
C++ |
4 |
Merge arrays |
C++ |
5 |
Min number |
C++ |
6 |
Permutations |
C++ |
7 |
Reorder numbers |
C++ |
# |
Title |
Solution |
1 |
Add numeric string |
C++ |
2 |
Allocate string |
C |
3 |
Anagram |
C++ |
4 |
Combinations |
C++ |
5 |
Delete char |
C++ |
6 |
Delete duplicate chars |
C++ |
7 |
Edit distance |
C++ |
8 |
First char appearing once |
C++ |
9 |
Numeric string |
C++ |
10 |
Palindrome number |
C++ |
11 |
Permutations |
C++ |
12 |
Regex |
C++ |
13 |
Replace blanks |
C++ |
14 |
Reverse words in a sentence |
C++ |
15 |
String path |
C++ |
# |
Title |
Solution |
1 |
Min in stack |
C++ |
2 |
Push pop order |
C++ |
3 |
Stream median |
C++ |
# |
Title |
Solution |
1 |
Clone complex list |
C++ |
2 |
Delete duplicates |
C++ |
3 |
Delete node |
C++ |
4 |
Kth node |
C++ |
5 |
Loops |
C++ |
6 |
Merge lists |
C++ |
7 |
Print reversely |
C++ |
8 |
Reverse list |
C++ |
9 |
Sort list |
C++ |
# |
Title |
Solution |
1 |
Fibonacci |
C++ |
2 |
Partially sorted matrix |
C++ |
3 |
Sorted matrix |
C++ |
# |
Title |
Solution |
1 |
Arrow and dot |
C++ |
2 |
Assigment operator |
C++ |
3 |
Objective C |
C |
# |
Title |
Solution |
1 |
Count ones |
C++ |
2 |
Modify numbers |
C++ |
3 |
Two missing numbers |
C++ |
4 |
Uniquely occuring numbers |
C++ |
# |
Title |
Solution |
1 |
Balance |
C++ |
2 |
Construct binary tree |
C++ |
3 |
Convert to list |
C++ |
4 |
Depth |
C++ |
5 |
Largest subtrees |
C++ |
6 |
Lowest ancestor |
C++ |
7 |
Nodes at k distance |
C++ |
8 |
Path |
C++ |
9 |
Print levels |
C++ |
10 |
Print zigzag |
C++ |
# |
Title |
Solution |
1 |
Coin change |
C++ |
2 |
Eight queens |
C++ |
3 |
Greatest sum subarrays |
C++ |
4 |
K least numbers |
C++ |
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Please make sure to update tests as appropriate.
MIT