first-timers-only
This issue is tagged 🕺 first-timers-only. It is only for people who have never contributed to open source before, and are looking for an easy way take their first steps.
Consider this your chance to dip your toe into the world of open-source, and get some bragging rights for writing code that makes drones fly, lets cars find charging stations, helps people and goods get from place to place, and more.
Find more first-timers-only issues from DAV Foundation here.
Thank you for your help ❤️
What is this project?
DAV (Decentralized Autonomous Vehicles) is a new foundation working to build an open-source infrastructure for autonomous vehicles (cars, drones, trucks, robots, and all the service providers around them) to communicate and transact with each other over blockchain.
As an organization that believes in building a large community of open-source contributors, we often create issues like this one to help people take their first few steps into the world of open source.
dav-js
This repo contains the DAV JavaScript SDK. This SDK allows developers to build applications and servers that connect to the DAV network. For example, allowing a drone to find charging stations, or an autonomous car to ask for traffic data.
How you can help
In order to foster a community that is welcoming for open source contributions, it is important for us to have good test coverage. And good tests are simple, readable tests.
Here is a good opportunity to update one of our tests.
The Issue
In the src/vessel-charging/BidParams.ts class, the serialize()
method is not returning our arguments correctly. provider
, manufacturer
and model
are being set to this.amenities
but they should be set to their associated arguments.
Currently:
public serialize() {
const formattedParams = super.serialize();
Object.assign(formattedParams, {
entranceLocation: this.entranceLocation,
exitLocation: this.exitLocation,
availableFrom: this.availableFrom,
availableUntil: this.availableUntil,
energySource: this.energySource,
amenities: this.amenities,
provider: this.amenities,
manufacturer: this.amenities,
model: this.amenities,
});
return formattedParams;
}
The Updates
Please update the test file src/vessel-charging/BidParams.test.ts to ensure amenities
, provider
, model
, manufacturer
are being handled correctly and update src/vessel-charging/BidParams.ts by passing the associated arguments to provider
, manufacturer
, model
.
1. Update the tests
The test should ensure amenities
, provider
, model
and manufacturer
are being passed through and returning correctly in the test file src/vessel-charging/BidParams.test.ts.
Please update this test by:
- Adding
Amenities
to the ./enums
import
import { EnergySources, Amenities } from './enums';
- Add arguments
amenities
, provider
, model
and manufacturer
to the instantiation of BidParams
const bidParams = new BidParams({
// existing params
amenities: [Amenities.Docking, Amenities.Grocery],
provider: 'Charging Co',
model: 'iCharger',
manufacturer: 'Vessel Chargers',
});
- Update serializedBidParams object with the arguments (
amenities
, provider
, model
and manufacturer
) passed above
const serializedBidParams: any = {
// existing properties
amenities: [Amenities.Docking, Amenities.Grocery],
provider: 'Charging Co',
model: 'iCharger',
manufacturer: 'Vessel Chargers',
};
After making your changes, run npm run jest
.
You should receive an error similar to below.
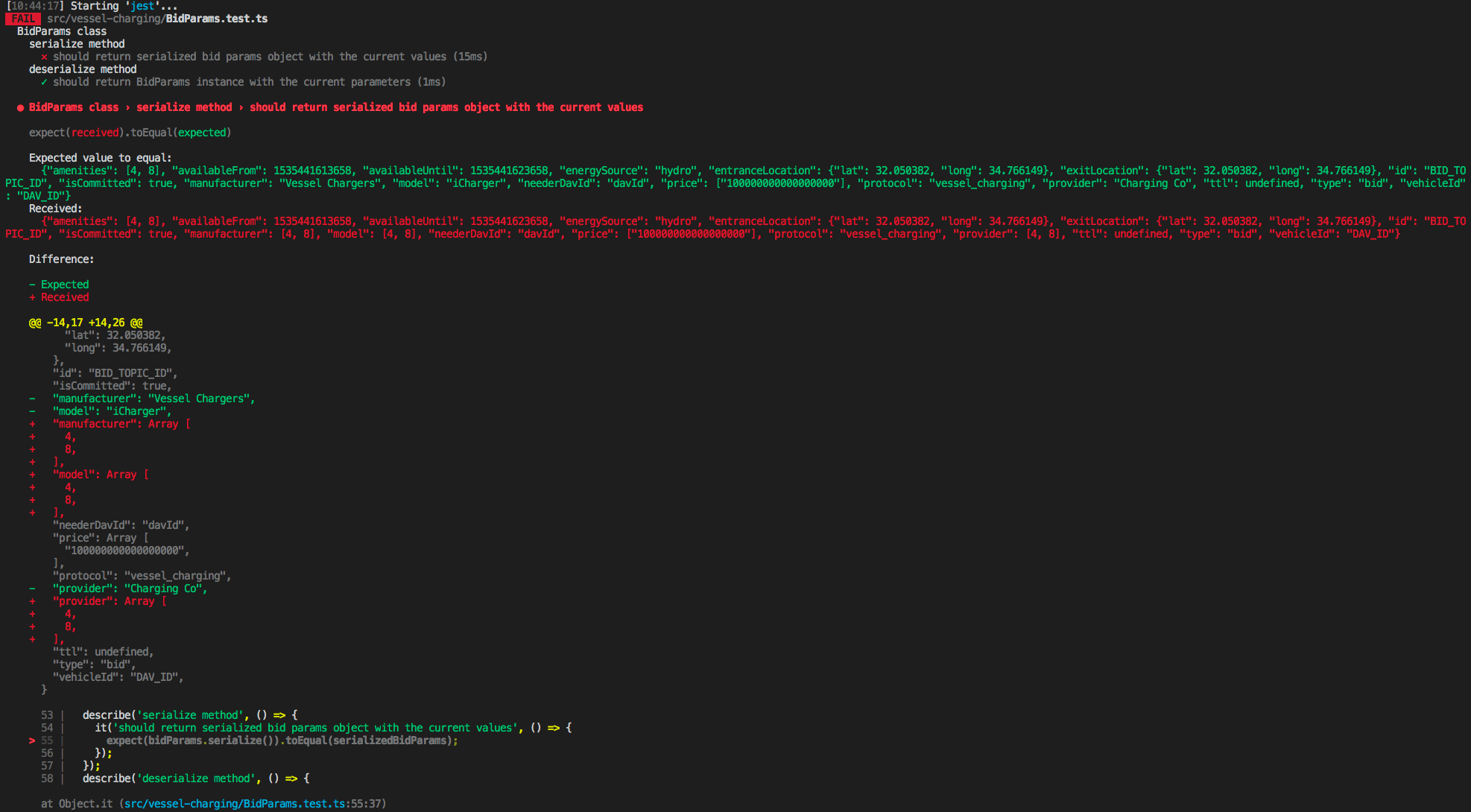
This is expected as the serialize()
method in src/vessel-charging/BidParams.ts is still returning provider
, manufacturer
and model
as this.amenities
.
The next update will fix this error.
2. Update serialize() in BidParams
The serialize()
method in src/vessel-charging/BidParams.ts should return the arguments associated with provider
, manufacturer
and model
.
- Update the
serialize()
method
public serialize() {
const formattedParams = super.serialize();
Object.assign(formattedParams, {
entranceLocation: this.entranceLocation,
exitLocation: this.exitLocation,
availableFrom: this.availableFrom,
availableUntil: this.availableUntil,
energySource: this.energySource,
amenities: this.amenities,
provider: this.provider,
manufacturer: this.manufacturer,
model: this.model,
});
return formattedParams;
}
After making your changes, run npm run jest
to make sure our tests are now passing.
Contributing to dav-js
Asking for help
We appreciate your effort in taking the time to work on this issue and help out the open source community and the foundation. If you need any help, feel free to ask below or in our gitter channel. We are always happy to help 😄