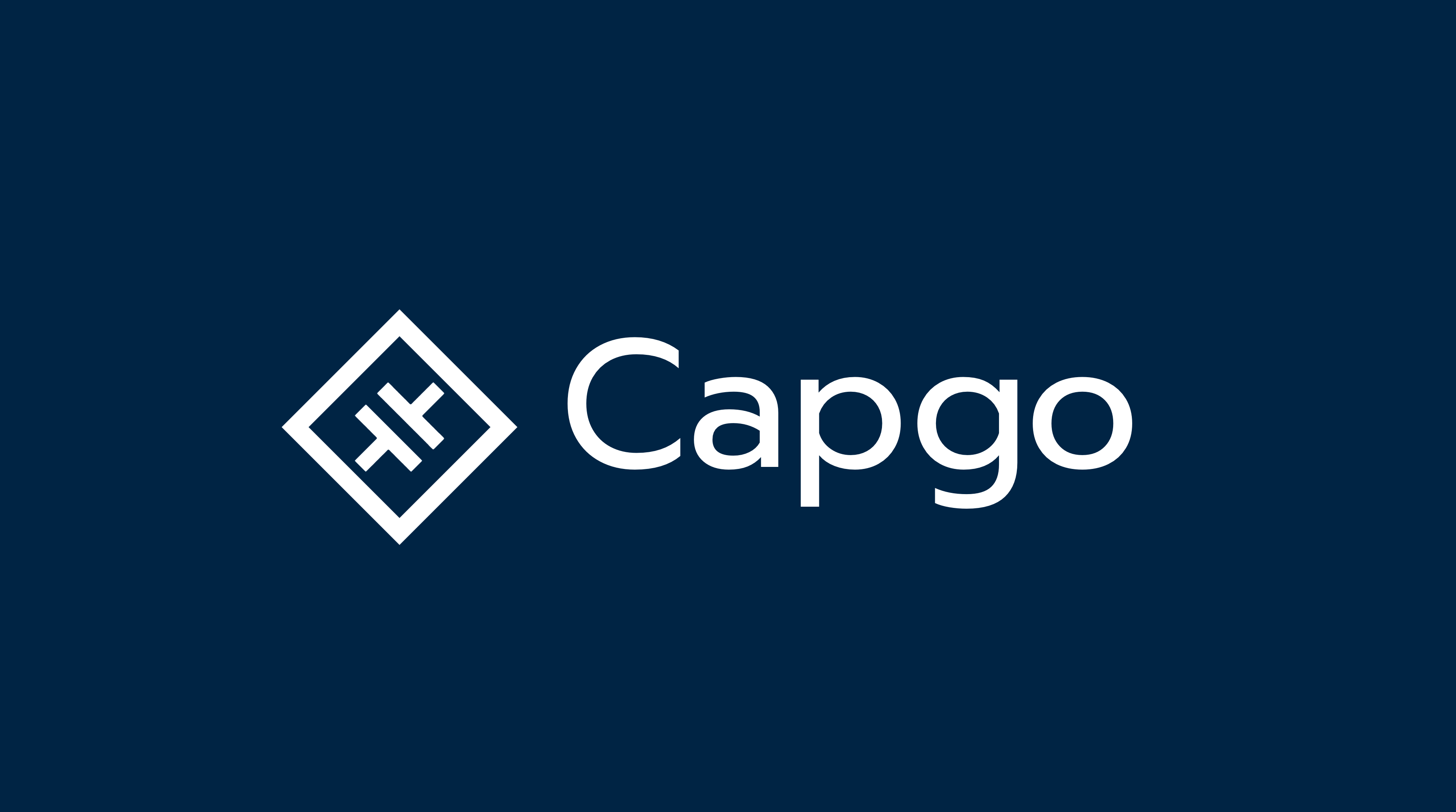
@capgo/native-audio
Capacitor plugin for playing sounds.
Capacitor plugin for native audio engine. Capacitor V6 - ✅ Support!
Click on video to see example 💥
Maintainer | GitHub | Social |
---|---|---|
Martin Donadieu | riderx | Telegram |
Mainteinance Status: Actively Maintained
All audio files must be with the rest of your source files.
First make your sound file end up in your builded code folder, example in folder BUILDFOLDER/assets/sounds/FILENAME.mp3
Then use it in preload like that assets/sounds/FILENAME.mp3
To use npm
npm install @capgo/native-audio
To use yarn
yarn add @capgo/native-audio
Sync native files
npx cap sync
On iOS, Android and Web, no further steps are needed.
No configuration required for this plugin.
Name | Android | iOS | Web |
---|---|---|---|
configure | ✅ | ✅ | ❌ |
preload | ✅ | ✅ | ✅ |
play | ✅ | ✅ | ✅ |
pause | ✅ | ✅ | ✅ |
resume | ✅ | ✅ | ✅ |
loop | ✅ | ✅ | ✅ |
stop | ✅ | ✅ | ✅ |
unload | ✅ | ✅ | ✅ |
setVolume | ✅ | ✅ | ✅ |
getDuration | ✅ | ✅ | ✅ |
getCurrentTime | ✅ | ✅ | ✅ |
isPlaying | ✅ | ✅ | ✅ |
import {NativeAudio} from '@capgo/native-audio'
/**
* This method will load more optimized audio files for background into memory.
* @param assetPath - relative path of the file, absolute url (file://) or remote url (https://)
* assetId - unique identifier of the file
* audioChannelNum - number of audio channels
* isUrl - pass true if assetPath is a `file://` url
* @returns void
*/
NativeAudio.preload({
assetId: "fire",
assetPath: "assets/sounds/fire.mp3",
audioChannelNum: 1,
isUrl: false
});
/**
* This method will play the loaded audio file if present in the memory.
* @param assetId - identifier of the asset
* @param time - (optional) play with seek. example: 6.0 - start playing track from 6 sec
* @returns void
*/
NativeAudio.play({
assetId: 'fire',
// time: 6.0 - seek time
});
/**
* This method will loop the audio file for playback.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.loop({
assetId: 'fire',
});
/**
* This method will stop the audio file if it's currently playing.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.stop({
assetId: 'fire',
});
/**
* This method will unload the audio file from the memory.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.unload({
assetId: 'fire',
});
/**
* This method will set the new volume for a audio file.
* @param assetId - identifier of the asset
* volume - numerical value of the volume between 0.1 - 1.0 default 1.0
* @returns void
*/
NativeAudio.setVolume({
assetId: 'fire',
volume: 0.4,
});
/**
* this method will get the duration of an audio file.
* only works if channels == 1
*/
NativeAudio.getDuration({
assetId: 'fire'
})
.then(result => {
console.log(result.duration);
})
/**
* this method will get the current time of a playing audio file.
* only works if channels == 1
*/
NativeAudio.getCurrentTime({
assetId: 'fire'
});
.then(result => {
console.log(result.currentTime);
})
/**
* This method will return false if audio is paused or not loaded.
* @param assetId - identifier of the asset
* @returns {isPlaying: boolean}
*/
NativeAudio.isPlaying({
assetId: 'fire'
})
.then(result => {
console.log(result.isPlaying);
})
configure(options: ConfigureOptions) => Promise<void>
Configure the audio player
Param | Type |
---|---|
options |
ConfigureOptions |
Since: 5.0.0
preload(options: PreloadOptions) => Promise<void>
Load an audio file
Param | Type |
---|---|
options |
PreloadOptions |
Since: 5.0.0
isPreloaded(options: PreloadOptions) => Promise<boolean>
Check if an audio file is preloaded
Param | Type |
---|---|
options |
PreloadOptions |
Returns: Promise<boolean>
Since: 6.1.0
play(options: { assetId: string; time?: number; delay?: number; }) => Promise<void>
Play an audio file
Param | Type |
---|---|
options |
{ assetId: string; time?: number; delay?: number; } |
Since: 5.0.0
pause(options: Assets) => Promise<void>
Pause an audio file
Param | Type |
---|---|
options |
Assets |
Since: 5.0.0
resume(options: Assets) => Promise<void>
Resume an audio file
Param | Type |
---|---|
options |
Assets |
Since: 5.0.0
loop(options: Assets) => Promise<void>
Stop an audio file
Param | Type |
---|---|
options |
Assets |
Since: 5.0.0
stop(options: Assets) => Promise<void>
Stop an audio file
Param | Type |
---|---|
options |
Assets |
Since: 5.0.0
unload(options: Assets) => Promise<void>
Unload an audio file
Param | Type |
---|---|
options |
Assets |
Since: 5.0.0
setVolume(options: { assetId: string; volume: number; }) => Promise<void>
Set the volume of an audio file
Param | Type |
---|---|
options |
{ assetId: string; volume: number; } |
Since: 5.0.0
setRate(options: { assetId: string; rate: number; }) => Promise<void>
Set the rate of an audio file
Param | Type |
---|---|
options |
{ assetId: string; rate: number; } |
Since: 5.0.0
getCurrentTime(options: { assetId: string; }) => Promise<{ currentTime: number; }>
Set the current time of an audio file
Param | Type |
---|---|
options |
{ assetId: string; } |
Returns: Promise<{ currentTime: number; }>
Since: 5.0.0
getDuration(options: Assets) => Promise<{ duration: number; }>
Get the duration of an audio file
Param | Type |
---|---|
options |
Assets |
Returns: Promise<{ duration: number; }>
Since: 5.0.0
isPlaying(options: Assets) => Promise<{ isPlaying: boolean; }>
Check if an audio file is playing
Param | Type |
---|---|
options |
Assets |
Returns: Promise<{ isPlaying: boolean; }>
Since: 5.0.0
addListener(eventName: "complete", listenerFunc: CompletedListener) => Promise<PluginListenerHandle>
Listen for complete event
Param | Type |
---|---|
eventName |
'complete' |
listenerFunc |
CompletedListener |
Returns: Promise<PluginListenerHandle>
Since: 5.0.0 return {@link CompletedEvent}
Prop | Type | Description |
---|---|---|
fade |
boolean |
Play the audio with Fade effect, only available for IOS |
focus |
boolean |
focus the audio with Audio Focus |
background |
boolean |
Play the audio in the background |
Prop | Type | Description |
---|---|---|
assetPath |
string |
Path to the audio file, relative path of the file, absolute url (file://) or remote url (https://) |
assetId |
string |
Asset Id, unique identifier of the file |
volume |
number |
Volume of the audio, between 0.1 and 1.0 |
audioChannelNum |
number |
Audio channel number, default is 1 |
isUrl |
boolean |
Is the audio file a URL, pass true if assetPath is a file:// url |
Prop | Type | Description |
---|---|---|
assetId |
string |
Asset Id, unique identifier of the file |
Prop | Type |
---|---|
remove |
() => Promise<void> |
Prop | Type | Description | Since |
---|---|---|---|
assetId |
string |
Emit when a play completes | 5.0.0 |
(state: CompletedEvent): void